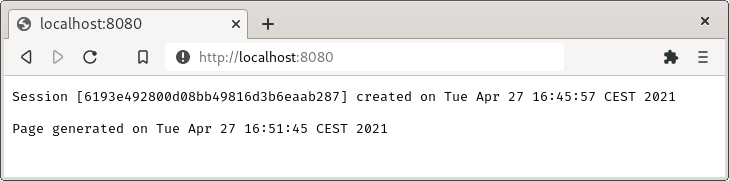
This document will show you how to store Vert.x Web Sessions in Infinispan.
You will build a Vert.x Web application that shows:
the session id and when the session was created
when the page was generated
The application fits in a single ServerVerticle
class.
A text editor or an IDE
Java 11 or higher
Maven or Gradle
An Infinispan cluster (or Docker)
The code of this project contains Maven and Gradle build files that are functionally equivalent.
Here is the content of the pom.xml
file you should be using:
pom.xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>io.vertx.howtos</groupId>
<artifactId>web-session-infinispan-howto</artifactId>
<version>1.0-SNAPSHOT</version>
<properties>
<vertx.version>4.4.0</vertx.version>
<maven.compiler.source>11</maven.compiler.source>
<maven.compiler.target>11</maven.compiler.target>
</properties>
<dependencyManagement>
<dependencies>
<dependency>
<groupId>io.vertx</groupId>
<artifactId>vertx-stack-depchain</artifactId>
<version>${vertx.version}</version>
<type>pom</type>
<scope>import</scope>
</dependency>
</dependencies>
</dependencyManagement>
<dependencies>
<dependency>
<groupId>io.vertx</groupId>
<artifactId>vertx-web-sstore-infinispan</artifactId>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<version>3.10.1</version>
<configuration>
<release>11</release>
</configuration>
</plugin>
<plugin>
<groupId>org.codehaus.mojo</groupId>
<artifactId>exec-maven-plugin</artifactId>
<version>1.5.0</version>
<configuration>
<mainClass>io.vertx.howtos.web.sessions.ServerVerticle</mainClass>
</configuration>
</plugin>
</plugins>
</build>
</project>
Assuming you use Gradle with the Kotlin DSL, here is what your build.gradle.kts
file should look like:
build.gradle.kts
plugins {
java
application
}
repositories {
mavenCentral()
}
java {
toolchain {
languageVersion.set(JavaLanguageVersion.of(11))
}
}
dependencies {
implementation(platform("io.vertx:vertx-stack-depchain:4.4.0"))
implementation("io.vertx:vertx-web-sstore-infinispan")
}
application {
mainClassName = "io.vertx.howtos.web.sessions.ServerVerticle"
}
tasks.wrapper {
gradleVersion = "7.6"
}
The Vert.x Web SessionStore
interface provides methods to read and modify session data.
The InfinispanSessionStore
implementation can be found in the io.vertx:vertx-web-sstore-infinispan
artifact.
Here is the code of the ServerVerticle
class:
package io.vertx.howtos.web.sessions;
import io.vertx.core.AbstractVerticle;
import io.vertx.core.Vertx;
import io.vertx.core.json.JsonArray;
import io.vertx.core.json.JsonObject;
import io.vertx.ext.web.Router;
import io.vertx.ext.web.Session;
import io.vertx.ext.web.handler.SessionHandler;
import io.vertx.ext.web.sstore.SessionStore;
import io.vertx.ext.web.sstore.infinispan.InfinispanSessionStore;
import java.util.Date;
public class ServerVerticle extends AbstractVerticle {
public static final String TEMPLATE = ""
+ "Session [%s] created on %s%n"
+ "%n"
+ "Page generated on %s%n";
@Override
public void start() {
Router router = Router.router(vertx);
JsonObject options = new JsonObject()
.put("servers", new JsonArray()
.add(new JsonObject()
.put("host", "localhost")
.put("port", 11222)
.put("username", "admin")
.put("password", "bar"))
);
SessionStore store = InfinispanSessionStore.create(vertx, options); // (1)
router.route().handler(SessionHandler.create(store)); // (2)
router.get("/").handler(ctx -> {
Session session = ctx.session();
session.computeIfAbsent("createdOn", s -> System.currentTimeMillis()); // (3)
String sessionId = session.id();
Date createdOn = new Date(session.<Long>get("createdOn"));
Date now = new Date();
ctx.end(String.format(TEMPLATE, sessionId, createdOn, now)); // (4)
});
vertx.createHttpServer()
.requestHandler(router)
.listen(8080);
}
public static void main(String[] args) {
Vertx vertx = Vertx.vertx();
vertx.deployVerticle(new ServerVerticle());
}
}
Create an InfinispanSessionStore
using configuration for your Infinispan Cluster (e.g. host, port and credentials).
Register the SessionHandler
, which manages Vert.x Web Sessions.
Put the session creation timestamp in the session data.
Create the response body and send it to the client.
If you’re not familiar with Infinispan, check out the introduction.
To run an Infinispan server on your machine with Docker, open your terminal and execute this:
docker run -p 11222:11222 -e USER="admin" -e PASS="bar" infinispan/server:13.0
Note
|
If you already have an Infinispan cluster running, don’t forget to update the configuration (host, port and credentials) in ServerVerticle .
|
The ServerVerticle
already has a main
method, so it can be used as-is to:
create a Vertx
context, then
deploy ServerVerticle
.
You can run the application from:
your IDE, by running the main
method from the ServerVerticle
class, or
with Maven: mvn compile exec:java
, or
with Gradle: ./gradlew run
(Linux, macOS) or gradle run
(Windows).
Browse to http://localhost:8080. If you request this page for the first time, the session creation time should be the same as the page generation time.
Now, let’s simulate failover to another web server by stopping the application and starting it again.
After the new web server has started, browse to http://localhost:8080 again. The session id and session creation time shouldn’t have changed (because session data has been persisted in Infinispan).
This document covered:
creating an InfinispanSessionStore
instance,
registering the Vert.x Web SessionHandler
,
modifying session data.
Last published: 2023-12-15 00:38:02 +0000.